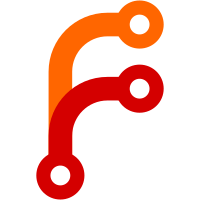
Instead of creating a new file for each player with their UUID as the name, we create a single file and store it all in a List. That List gets converted to a UUID -> SoulNetwork map when read from the file. MigrateNetworkDataHandler is used to migrate players from the old system to the new one. It reads both data files and sets the LP of the new network to the LP of the old network (if the old network is larger). Once conversion is done, we delete the old file so that it doesn't happen again and overwrite player progress. This is an API breaking change due to an import change.
65 lines
1.8 KiB
Java
65 lines
1.8 KiB
Java
package WayofTime.bloodmagic.api.saving;
|
|
|
|
import WayofTime.bloodmagic.api.util.helper.PlayerHelper;
|
|
import net.minecraft.entity.player.EntityPlayer;
|
|
import net.minecraft.nbt.NBTTagCompound;
|
|
import net.minecraft.nbt.NBTTagList;
|
|
import net.minecraft.world.WorldSavedData;
|
|
|
|
import java.util.*;
|
|
|
|
public class BMWorldSavedData extends WorldSavedData
|
|
{
|
|
public static final String ID = "BloodMagic-SoulNetworks";
|
|
|
|
private Map<UUID, SoulNetwork> soulNetworks = new HashMap<UUID, SoulNetwork>();
|
|
|
|
public BMWorldSavedData(String id)
|
|
{
|
|
super(id);
|
|
}
|
|
|
|
public BMWorldSavedData()
|
|
{
|
|
this(ID);
|
|
}
|
|
|
|
public SoulNetwork getNetwork(EntityPlayer player)
|
|
{
|
|
return getNetwork(PlayerHelper.getUUIDFromPlayer(player));
|
|
}
|
|
|
|
public SoulNetwork getNetwork(UUID playerId)
|
|
{
|
|
if (!soulNetworks.containsKey(playerId))
|
|
soulNetworks.put(playerId, SoulNetwork.newEmpty(playerId).setParent(this));
|
|
return soulNetworks.get(playerId);
|
|
}
|
|
|
|
@Override
|
|
public void readFromNBT(NBTTagCompound tagCompound)
|
|
{
|
|
NBTTagList networkData = tagCompound.getTagList("networkData", 10);
|
|
|
|
for (int i = 0; i < networkData.tagCount(); i++)
|
|
{
|
|
NBTTagCompound data = networkData.getCompoundTagAt(i);
|
|
SoulNetwork network = SoulNetwork.fromNBT(data);
|
|
network.setParent(this);
|
|
soulNetworks.put(network.getPlayerId(), network);
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public NBTTagCompound writeToNBT(NBTTagCompound tagCompound)
|
|
{
|
|
NBTTagList networkData = new NBTTagList();
|
|
for (SoulNetwork soulNetwork : soulNetworks.values())
|
|
networkData.appendTag(soulNetwork.serializeNBT());
|
|
|
|
tagCompound.setTag("networkData", networkData);
|
|
|
|
return tagCompound;
|
|
}
|
|
}
|