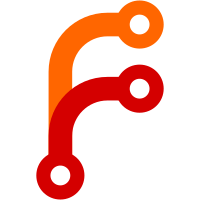
I redone where the items/blocsks are stored and how the configs are handled to clean up it and give space. You can change the config line to AWWayofTime if you want to keep the compatibility with old configs. Now you reference the blocks from the ModBlocks and Items from the ModItems.
93 lines
2.2 KiB
Java
93 lines
2.2 KiB
Java
package thaumcraft.api.aspects;
|
|
|
|
import net.minecraftforge.common.ForgeDirection;
|
|
|
|
/**
|
|
* @author Azanor
|
|
* This interface is used by tiles that use or transport vis.
|
|
* Only tiles that implement this interface will be able to connect to vis conduits or other thaumic devices
|
|
*/
|
|
public interface IEssentiaTransport {
|
|
/**
|
|
* Is this tile able to connect to other vis users/sources on the specified side?
|
|
*
|
|
* @param face
|
|
* @return
|
|
*/
|
|
public boolean isConnectable(ForgeDirection face);
|
|
|
|
/**
|
|
* Is this side used to input essentia?
|
|
*
|
|
* @param face
|
|
* @return
|
|
*/
|
|
boolean canInputFrom(ForgeDirection face);
|
|
|
|
/**
|
|
* Is this side used to output essentia?
|
|
*
|
|
* @param face
|
|
* @return
|
|
*/
|
|
boolean canOutputTo(ForgeDirection face);
|
|
|
|
// /**
|
|
// * Can this tile act as a source of vis?
|
|
// * @return
|
|
// */
|
|
// public boolean isVisSource();
|
|
//
|
|
// /**
|
|
// * Is this tile a conduit that transports vis?
|
|
// * @return
|
|
// */
|
|
// public boolean isVisConduit();
|
|
|
|
/**
|
|
* Sets the amount of suction this block will apply
|
|
*
|
|
* @param suction
|
|
*/
|
|
public void setSuction(AspectList suction);
|
|
|
|
/**
|
|
* Sets the amount of suction this block will apply
|
|
*
|
|
* @param suction
|
|
*/
|
|
public void setSuction(Aspect aspect, int amount);
|
|
|
|
/**
|
|
* Returns the amount of suction this block is applying.
|
|
*
|
|
* @param loc the location from where the suction is being checked
|
|
* @return
|
|
*/
|
|
public AspectList getSuction(ForgeDirection face);
|
|
|
|
/**
|
|
* remove the specified amount of vis from this transport tile
|
|
*
|
|
* @param suction
|
|
* @return how much was actually taken
|
|
*/
|
|
public int takeVis(Aspect aspect, int amount);
|
|
|
|
public AspectList getEssentia(ForgeDirection face);
|
|
|
|
/**
|
|
* Essentia will not be drawn from this container unless the suction exceeds this amount.
|
|
*
|
|
* @return the amount
|
|
*/
|
|
public int getMinimumSuction();
|
|
|
|
/**
|
|
* Return true if you want the conduit to extend a little further into the block.
|
|
* Used by jars and alembics that have smaller than normal hitboxes
|
|
*
|
|
* @return
|
|
*/
|
|
boolean renderExtendedTube();
|
|
}
|