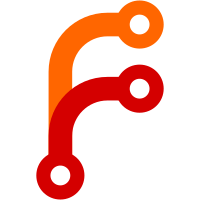
I redone where the items/blocsks are stored and how the configs are handled to clean up it and give space. You can change the config line to AWWayofTime if you want to keep the compatibility with old configs. Now you reference the blocks from the ModBlocks and Items from the ModItems.
80 lines
2.1 KiB
Java
80 lines
2.1 KiB
Java
package thaumcraft.api;
|
|
|
|
import cpw.mods.fml.common.FMLLog;
|
|
import net.minecraft.block.Block;
|
|
import net.minecraft.item.Item;
|
|
import net.minecraft.item.ItemStack;
|
|
|
|
/**
|
|
* @author Azanor
|
|
* <p/>
|
|
* This is used to gain access to the items in my mod.
|
|
* I only give some examples and it will probably still
|
|
* require a bit of work for you to get hold of everything you need.
|
|
*/
|
|
public class ItemApi {
|
|
public static ItemStack getItem(String itemString, int meta)
|
|
{
|
|
ItemStack item = null;
|
|
|
|
try
|
|
{
|
|
String itemClass = "thaumcraft.common.config.ConfigItems";
|
|
Object obj = Class.forName(itemClass).getField(itemString).get(null);
|
|
|
|
if (obj instanceof Item)
|
|
{
|
|
item = new ItemStack((Item) obj, 1, meta);
|
|
} else if (obj instanceof ItemStack)
|
|
{
|
|
item = (ItemStack) obj;
|
|
}
|
|
} catch (Exception ex)
|
|
{
|
|
FMLLog.warning("[Thaumcraft] Could not retrieve item identified by: " + itemString);
|
|
}
|
|
|
|
return item;
|
|
}
|
|
|
|
public static ItemStack getBlock(String itemString, int meta)
|
|
{
|
|
ItemStack item = null;
|
|
|
|
try
|
|
{
|
|
String itemClass = "thaumcraft.common.config.ConfigBlocks";
|
|
Object obj = Class.forName(itemClass).getField(itemString).get(null);
|
|
|
|
if (obj instanceof Block)
|
|
{
|
|
item = new ItemStack((Block) obj, 1, meta);
|
|
} else if (obj instanceof ItemStack)
|
|
{
|
|
item = (ItemStack) obj;
|
|
}
|
|
} catch (Exception ex)
|
|
{
|
|
FMLLog.warning("[Thaumcraft] Could not retrieve block identified by: " + itemString);
|
|
}
|
|
|
|
return item;
|
|
}
|
|
|
|
/**
|
|
*
|
|
* Some examples
|
|
*
|
|
* Casting Wands:
|
|
* itemWandCasting
|
|
*
|
|
* Resources:
|
|
* itemEssence, itemWispEssence, itemResource, itemShard, itemNugget,
|
|
* itemNuggetChicken, itemNuggetBeef, itemNuggetPork, itemTripleMeatTreat
|
|
*
|
|
* Research:
|
|
* itemResearchNotes, itemInkwell, itemThaumonomicon
|
|
*
|
|
*/
|
|
|
|
}
|