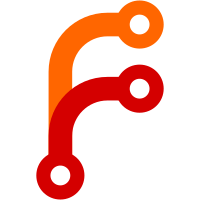
I redone where the items/blocsks are stored and how the configs are handled to clean up it and give space. You can change the config line to AWWayofTime if you want to keep the compatibility with old configs. Now you reference the blocks from the ModBlocks and Items from the ModItems.
52 lines
1.4 KiB
Java
52 lines
1.4 KiB
Java
package WayofTime.alchemicalWizardry.common.alchemy;
|
|
|
|
import net.minecraft.item.ItemBlock;
|
|
import net.minecraft.item.ItemStack;
|
|
|
|
public class AlchemyPotionHandlerComponent {
|
|
private ItemStack itemStack;
|
|
private int potionID;
|
|
private int tickDuration;
|
|
|
|
public AlchemyPotionHandlerComponent(ItemStack itemStack, int potionID, int tickDuration)
|
|
{
|
|
this.itemStack = itemStack;
|
|
this.potionID = potionID;
|
|
this.tickDuration = tickDuration;
|
|
}
|
|
|
|
public boolean compareItemStack(ItemStack comparedStack)
|
|
{
|
|
if (comparedStack != null && itemStack != null)
|
|
{
|
|
if (comparedStack.getItem() instanceof ItemBlock)
|
|
{
|
|
if (itemStack.getItem() instanceof ItemBlock)
|
|
{
|
|
return comparedStack.itemID == itemStack.itemID && comparedStack.getItemDamage() == itemStack.getItemDamage();
|
|
}
|
|
} else if (!(itemStack.getItem() instanceof ItemBlock))
|
|
{
|
|
return comparedStack.itemID == itemStack.itemID && comparedStack.getItemDamage() == itemStack.getItemDamage();
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
public ItemStack getItemStack()
|
|
{
|
|
return itemStack;
|
|
}
|
|
|
|
public int getPotionID()
|
|
{
|
|
return this.potionID;
|
|
}
|
|
|
|
public int getTickDuration()
|
|
{
|
|
return this.tickDuration;
|
|
}
|
|
}
|