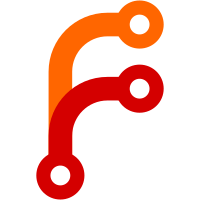
All old configs must be deleted for this to work properly. Since the rest
of the update is filled with world breaking changes, this should be fine.
Also some mapping updates
(cherry picked from commit d587a8c
)
83 lines
2.9 KiB
Java
83 lines
2.9 KiB
Java
package WayofTime.bloodmagic.item.soul;
|
|
|
|
import WayofTime.bloodmagic.BloodMagic;
|
|
import WayofTime.bloodmagic.client.IVariantProvider;
|
|
import WayofTime.bloodmagic.entity.projectile.EntitySoulSnare;
|
|
import WayofTime.bloodmagic.util.helper.TextHelper;
|
|
import net.minecraft.client.util.ITooltipFlag;
|
|
import net.minecraft.creativetab.CreativeTabs;
|
|
import net.minecraft.entity.player.EntityPlayer;
|
|
import net.minecraft.init.SoundEvents;
|
|
import net.minecraft.item.Item;
|
|
import net.minecraft.item.ItemStack;
|
|
import net.minecraft.util.*;
|
|
import net.minecraft.world.World;
|
|
import net.minecraftforge.fml.relauncher.Side;
|
|
import net.minecraftforge.fml.relauncher.SideOnly;
|
|
import org.apache.commons.lang3.tuple.ImmutablePair;
|
|
import org.apache.commons.lang3.tuple.Pair;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.Arrays;
|
|
import java.util.List;
|
|
|
|
public class ItemSoulSnare extends Item implements IVariantProvider {
|
|
public static String[] names = {"base"};
|
|
|
|
public ItemSoulSnare() {
|
|
super();
|
|
|
|
setUnlocalizedName(BloodMagic.MODID + ".soulSnare.");
|
|
setCreativeTab(BloodMagic.TAB_BM);
|
|
setHasSubtypes(true);
|
|
setMaxStackSize(16);
|
|
}
|
|
|
|
@Override
|
|
public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand hand) {
|
|
ItemStack stack = playerIn.getHeldItem(hand);
|
|
if (!playerIn.capabilities.isCreativeMode) {
|
|
stack.shrink(1);
|
|
}
|
|
|
|
worldIn.playSound(null, playerIn.posX, playerIn.posY, playerIn.posZ, SoundEvents.ENTITY_SNOWBALL_THROW, SoundCategory.NEUTRAL, 0.5F, 0.4F / (itemRand.nextFloat() * 0.4F + 0.8F));
|
|
|
|
if (!worldIn.isRemote) {
|
|
EntitySoulSnare snare = new EntitySoulSnare(worldIn, playerIn);
|
|
snare.shoot(playerIn, playerIn.rotationPitch, playerIn.rotationYaw, 0.0F, 1.5F, 1.0F);
|
|
worldIn.spawnEntity(snare);
|
|
}
|
|
|
|
return new ActionResult<ItemStack>(EnumActionResult.SUCCESS, stack);
|
|
}
|
|
|
|
@Override
|
|
public String getUnlocalizedName(ItemStack stack) {
|
|
return super.getUnlocalizedName(stack) + names[stack.getItemDamage()];
|
|
}
|
|
|
|
@Override
|
|
@SideOnly(Side.CLIENT)
|
|
public void getSubItems(CreativeTabs creativeTab, NonNullList<ItemStack> list) {
|
|
if (!isInCreativeTab(creativeTab))
|
|
return;
|
|
|
|
for (int i = 0; i < names.length; i++)
|
|
list.add(new ItemStack(this, 1, i));
|
|
}
|
|
|
|
@Override
|
|
@SideOnly(Side.CLIENT)
|
|
public void addInformation(ItemStack stack, World world, List<String> tooltip, ITooltipFlag flag) {
|
|
tooltip.addAll(Arrays.asList(TextHelper.cutLongString(TextHelper.localizeEffect("tooltip.bloodmagic.soulSnare.desc"))));
|
|
|
|
super.addInformation(stack, world, tooltip, flag);
|
|
}
|
|
|
|
@Override
|
|
public List<Pair<Integer, String>> getVariants() {
|
|
List<Pair<Integer, String>> ret = new ArrayList<Pair<Integer, String>>();
|
|
ret.add(new ImmutablePair<Integer, String>(0, "type=soulsnare"));
|
|
return ret;
|
|
}
|
|
}
|