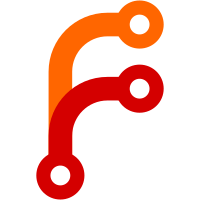
This DOES NOT BUILD. Do not even attempt. Almost everything has been ported besides the block/item packages. Then it's a matter of testing what broke.
43 lines
1.1 KiB
Java
43 lines
1.1 KiB
Java
package WayofTime.bloodmagic.block;
|
|
|
|
import WayofTime.bloodmagic.api.Constants;
|
|
import WayofTime.bloodmagic.tile.routing.TileItemRoutingNode;
|
|
import WayofTime.bloodmagic.tile.routing.TileRoutingNode;
|
|
import net.minecraft.block.state.IBlockState;
|
|
import net.minecraft.tileentity.TileEntity;
|
|
import net.minecraft.util.BlockPos;
|
|
import net.minecraft.world.World;
|
|
|
|
public class BlockItemRoutingNode extends BlockRoutingNode
|
|
{
|
|
public BlockItemRoutingNode()
|
|
{
|
|
super();
|
|
|
|
setUnlocalizedName(Constants.Mod.MODID + ".itemRouting");
|
|
setRegistryName(Constants.BloodMagicBlock.ITEM_ROUTING_NODE.getRegName());
|
|
}
|
|
|
|
@Override
|
|
public int getRenderType()
|
|
{
|
|
return 3;
|
|
}
|
|
|
|
@Override
|
|
public TileEntity createNewTileEntity(World worldIn, int meta)
|
|
{
|
|
return new TileItemRoutingNode();
|
|
}
|
|
|
|
@Override
|
|
public void breakBlock(World world, BlockPos pos, IBlockState state)
|
|
{
|
|
TileEntity tile = world.getTileEntity(pos);
|
|
if (tile instanceof TileRoutingNode)
|
|
{
|
|
((TileRoutingNode) tile).removeAllConnections();
|
|
}
|
|
super.breakBlock(world, pos, state);
|
|
}
|
|
}
|