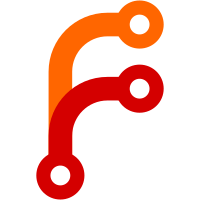
* Initial stab at API structuring * Throwing all the things into the API* Eliminated all internal imports Also added some helpful comments *except for the ritual stuff * Reducing the API Threw back the altar/incense/unnecessary items to main Added in a functional API instance * API cleanup Removing all the unnecessities Smushed and vaporized some redundant recipe stuffs * Made API dummy instances Refactor packaging
53 lines
No EOL
1.6 KiB
Java
53 lines
No EOL
1.6 KiB
Java
package wayoftime.bloodmagic.common.item;
|
|
|
|
import java.util.List;
|
|
|
|
import net.minecraft.client.util.ITooltipFlag;
|
|
import net.minecraft.entity.Entity;
|
|
import net.minecraft.entity.player.PlayerEntity;
|
|
import net.minecraft.item.Item;
|
|
import net.minecraft.item.ItemStack;
|
|
import net.minecraft.util.text.ITextComponent;
|
|
import net.minecraft.util.text.TranslationTextComponent;
|
|
import net.minecraft.world.World;
|
|
import net.minecraftforge.api.distmarker.Dist;
|
|
import net.minecraftforge.api.distmarker.OnlyIn;
|
|
import wayoftime.bloodmagic.BloodMagic;
|
|
import wayoftime.bloodmagic.api.compat.IDemonWillViewer;
|
|
import wayoftime.bloodmagic.util.handler.event.GenericHandler;
|
|
|
|
public class ItemDemonWillGauge extends Item implements IDemonWillViewer
|
|
{
|
|
public ItemDemonWillGauge()
|
|
{
|
|
super(new Item.Properties().maxStackSize(1).group(BloodMagic.TAB));
|
|
}
|
|
|
|
@Override
|
|
@OnlyIn(Dist.CLIENT)
|
|
public void addInformation(ItemStack stack, World world, List<ITextComponent> tooltip, ITooltipFlag flag)
|
|
{
|
|
tooltip.add(new TranslationTextComponent("tooltip.bloodmagic.willGauge"));
|
|
}
|
|
|
|
@Override
|
|
public boolean canSeeDemonWillAura(World world, ItemStack stack, PlayerEntity player)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
@Override
|
|
public int getDemonWillAuraResolution(World world, ItemStack stack, PlayerEntity player)
|
|
{
|
|
return 100;
|
|
}
|
|
|
|
@Override
|
|
public void inventoryTick(ItemStack stack, World worldIn, Entity entityIn, int itemSlot, boolean isSelected)
|
|
{
|
|
if (entityIn instanceof PlayerEntity && entityIn.ticksExisted % 50 == 0)
|
|
{
|
|
GenericHandler.sendPlayerDemonWillAura((PlayerEntity) entityIn);
|
|
}
|
|
}
|
|
} |