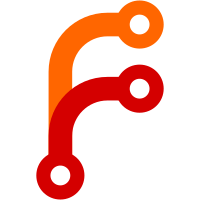
* Reinstated Compression sigil. - does not compress wooden planks into crafting tables - searches for reversible 3x3/2x2 recipes with a single material type - probably has a lot of redundant stuff and looks silly - uses try/catch (might want to find a different approach, some people scoff at this) - should probably have spent the night sleeping, I'm taking exams tomorrow and will probably sleep the whole day through. * Learned how to properly handle the "NullPointerException"-situation. * Update BaseCompressionHandler.java * Update CompressionRegistry.java * Removed (almost) all code comments (only a one-liner remains that serves as pointer to a completly commented-out class (StorageBlockCraftingRecipeAssimilator)). Made methods and variables for long function calls to be more efficient. Fixed an oversight that resulted in a NullPointerException after removing redundant checks that were made to prevent exactly that. Rearranged and reformatted code. * corrected something that could be considered a typo but was an oversight * This should be it. An Array should be more efficient but you can correct me if I'm wrong. In either case it does what it is supposed to do. * Fixed. Needed a seperate inventory for the reversible check (or clear the previously used one, but then I'd had to repopulate again and that would just have been messy) * Forgot one of my lines. * Fix and cleanup. Could definitely clean more but that should suffice for now.
52 lines
1.4 KiB
Java
52 lines
1.4 KiB
Java
package WayofTime.bloodmagic.compress;
|
|
|
|
import net.minecraft.item.ItemStack;
|
|
import net.minecraft.world.World;
|
|
|
|
public class BaseCompressionHandler extends CompressionHandler {
|
|
private final ItemStack required;
|
|
private final ItemStack result;
|
|
private final int leftover;
|
|
|
|
public BaseCompressionHandler(ItemStack input, ItemStack output, int remainder) {
|
|
super();
|
|
this.required = input;
|
|
this.result = output;
|
|
this.leftover = remainder;
|
|
}
|
|
|
|
public ItemStack getResultStack() {
|
|
return this.result.copy();
|
|
}
|
|
|
|
public ItemStack getRequiredStack() {
|
|
return this.required.copy();
|
|
}
|
|
|
|
@Override
|
|
public ItemStack compressInventory(ItemStack[] inv, World world) {
|
|
int remaining = this.getRemainingNeeded(inv);
|
|
if (remaining <= 0) {
|
|
this.drainInventory(inv);
|
|
return this.getResultStack();
|
|
}
|
|
|
|
return ItemStack.EMPTY;
|
|
}
|
|
|
|
public int getRemainingNeeded(ItemStack[] inv) {
|
|
int needed = this.required.getCount();
|
|
int kept = this.getLeftover();
|
|
return iterateThroughInventory(this.required, kept, inv, needed, true);
|
|
}
|
|
|
|
public int drainInventory(ItemStack[] inv) {
|
|
int needed = this.required.getCount();
|
|
int kept = this.getLeftover();
|
|
return iterateThroughInventory(this.required, kept, inv, needed, true);
|
|
}
|
|
|
|
public int getLeftover() {
|
|
return this.leftover;
|
|
}
|
|
} |