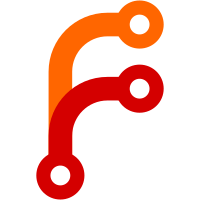
Need to work on how the EntitySpellProjectile saves its data. Does not work properly. Perhaps it was not registered?
73 lines
2.1 KiB
Java
73 lines
2.1 KiB
Java
package WayofTime.alchemicalWizardry.common.tileEntity;
|
|
|
|
import java.util.List;
|
|
|
|
import net.minecraft.entity.player.EntityPlayer;
|
|
import net.minecraft.item.ItemStack;
|
|
import net.minecraft.util.ChatMessageComponent;
|
|
import net.minecraft.world.World;
|
|
import WayofTime.alchemicalWizardry.common.spell.complex.SpellParadigm;
|
|
import WayofTime.alchemicalWizardry.common.spell.complex.SpellParadigmMelee;
|
|
import WayofTime.alchemicalWizardry.common.spell.complex.SpellParadigmProjectile;
|
|
import WayofTime.alchemicalWizardry.common.spell.complex.SpellParadigmSelf;
|
|
|
|
public class TESpellParadigmBlock extends TESpellBlock
|
|
{
|
|
public SpellParadigm getSpellParadigm()
|
|
{
|
|
int meta = worldObj.getBlockMetadata(xCoord, yCoord, zCoord);
|
|
switch(meta)
|
|
{
|
|
case 0: return new SpellParadigmProjectile();
|
|
case 1: return new SpellParadigmSelf();
|
|
case 2: return new SpellParadigmMelee();
|
|
}
|
|
return new SpellParadigmSelf();
|
|
}
|
|
|
|
@Override
|
|
protected void applySpellChange(SpellParadigm parad)
|
|
{
|
|
return;
|
|
}
|
|
|
|
public boolean canInputRecieve()
|
|
{
|
|
return false;
|
|
}
|
|
|
|
public void castSpell(World world, EntityPlayer entity, ItemStack spellCasterStack)
|
|
{
|
|
SpellParadigm parad = this.getSpellParadigm();
|
|
this.modifySpellParadigm(parad);
|
|
// if(parad instanceof SpellParadigmSelf)
|
|
// {
|
|
// List<String> stringList = parad.effectList;
|
|
// SpellParadigmSelf spellParadSelf = SpellParadigmSelf.getParadigmForStringArray(stringList);
|
|
// for(String str : stringList)
|
|
// {
|
|
// ChatMessageComponent chat = new ChatMessageComponent();
|
|
// chat.addText(str);
|
|
// entity.sendChatToPlayer(chat);
|
|
// }
|
|
// spellParadSelf.castSpell(world, entity, spellCasterStack);
|
|
// }
|
|
// else if(parad instanceof SpellParadigmProjectile)
|
|
// {
|
|
// List<String> stringList = parad.effectList;
|
|
// SpellParadigmProjectile spellParadSelf = SpellParadigmProjectile.getParadigmForStringArray(stringList);
|
|
// for(String str : stringList)
|
|
// {
|
|
// ChatMessageComponent chat = new ChatMessageComponent();
|
|
// chat.addText(str);
|
|
// entity.sendChatToPlayer(chat);
|
|
// }
|
|
// spellParadSelf.castSpell(world, entity, spellCasterStack);
|
|
// }else
|
|
{
|
|
parad.castSpell(world, entity, spellCasterStack);
|
|
}
|
|
|
|
}
|
|
}
|